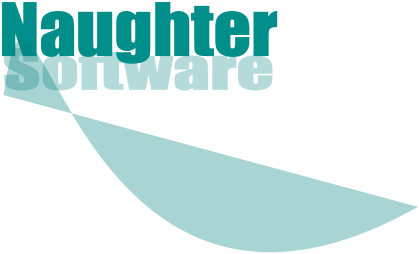
Welcome to 8055N++, a collection of Open Source C++ classes which
encapsulates the K8055,
K8055N,
WPI110N,
WSI8055N,
VM110 &
VM110N USB Experiment Interface boards
from Velleman / Whadda using the Windows HID APIs.
The code below shows a simple example to connect to the board and blink all
the digital outputs on the board:
_tprintf(_T("Enumerating K8055, K8055N, VM110 & VM110N HID devices on
this computer\n"));
HID::StringArray deviceNames;
bool bSuccess =
HID::C8055NDevice::Enumerate(deviceNames);
HID::String sDevicePath;
for
(const auto& device : deviceNames)
{
sDevicePath = device;
_tprintf(_T(" Found device path of %s\n"), device.c_str());
}
if
(sDevicePath.length() == 0)
{
_tprintf(_T(" No devices found\n"));
return 1;
}
HID::C8055NDevice device;
device.Open(sDevicePath.c_str());
int nCycleDigitalChannel{1};
while
(true)
{
device.ClearDigitalChannel(nCycleDigitalChannel);
Sleep(100);
nCycleDigitalChannel++;
if (nCycleDigitalChannel
== 9)
nCycleDigitalChannel = 1;
device.SetDigitalChannel(nCycleDigitalChannel);
}
The complete list of classes which 8055N+ provides are:
- HID::C8055NDevice which provides a C++ class encapsulation of the
functionality of the boards. This class uses the author's
HIDWrappers to talk
to the boards..
- HID::C8055NClientDevice which provides a wrapper for the K8055D.DLL
which is the primary API interface to the boards. This class can talk to
either the Vellerman DLL or the author's reimplementation. This class hides
the details of using LoadLibrary / GetProcAddress.
- HID::C8055NClientDevice2 This class is used to implement the K8055D.DLL
implementation included in 8055N++. Client code can also use this instead of
HID::C8055NClientDevice to talk to HID::C8055NDevice without the need to go
through the K8055D.DLL.
Features
- Supports all the K8055, K8055N, VM110 & VM110N USB Experiment Interface
board variants. This includes the old USB protocol used by the K8055 and
VM110 boards and the newer USB protocol supported by the K8055N and VM110N
boards.
- Encapsulates all the
functionality exposed by the boards as of v5.0.0.4 of the Velleman
K8055D.DLL.
- Functionality is implemented directly using C++ without the need to go
through the Velleman K8055D.DLL if desired.
- Implements a compatible version of the Velleman DLL for existing
applications which use the Velleman DLL. Binaries for this DLL are provided
in x86, x64, ARM32 and ARM64 variants for Windows in the download.
- The classes have no external dependencies on other libraries such as ATL or
MFC.
- Provides a full reimplementation of the Velleman demo application in C++
MFC.
- The reimplementation of the Velleman demo application can use either the
Velleman K8055D.DLL, the author's replacement K8055D.DLL or can call the
8055N+ classes directly, to talk to the boards.
The enclosed zip file contains the
8055N++ source code and a VC 2022 solution which
demonstrates most of the classes functionality.
Copyright
- You are allowed to include the source code in any product (commercial, shareware,
freeware or otherwise) when your product is released in binary form.
- You are allowed to modify the source code in any way you want except you
cannot modify the copyright details at the top of each module.
- If you want to distribute source code with your application, then you are
only allowed to distribute versions released by the author. This is to maintain
a single distribution point for the source code.
Updates
v1.0 (8 April 2023)