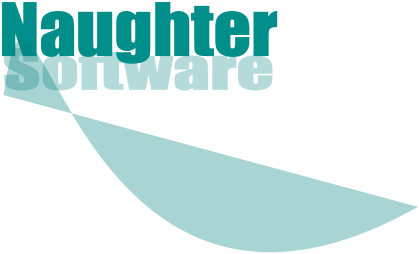
CFTPTransferDlg provides an MFC dialog which performs FTP uploads and downloads
similar to the old Internet Explorer download dialog as shown below:
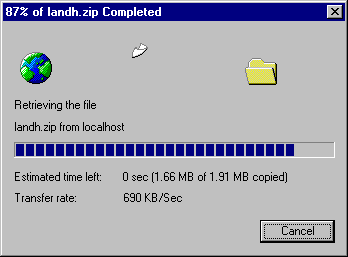
To use CFTPTransferDlg in your project simply include FTPTransferDlg.cpp/h and
FTPTransferer.cpp/h from the test application in your application and #include "FTPTransferDlg.h"
in whichever files you want to use the class in. You should also copy over all the "IDS_FTPTRANSFER_.."
string resources, the IDD_FTPTRANSFER dialog resource and the IDR_FTPTRANSFER_ANIMATION "avi"
resource to your application. Then to bring up the dialog to upload / download a
specific file, just use some code like the following:
CFTPTransferDlg dlg;
dlg.m_sServer = _T("ftp.some-site.com");
dlg.m_sRemoteFile = _T("/somefile.ext");
dlg.m_sLocalFile = _T("c:\\somfile.ext");
dlg.m_bDownload = TRUE;
dlg.m_bBinary = TRUE;
if (dlg.DoModal() == IDOK)
AfxMessageBox("File
was downloaded successfully");
Alternatively you can use the CFTPTransferer class (which CFTPTransferDlg uses
internally) in a synchronous non-UI manner as follows:
CFTPTransferer transfer;
transfer.m_sServer = _T("ftp.some-site.com");
transfer.m_sRemoteFile
= _T("/somefile.ext");
transfer.m_sLocalFile = _T("c:\\somfile.ext");
transfer.m_bDownload = TRUE;
transfer.Transfer();
The enclosed zip file contains source
code for the classes and also includes a VC 2017 project to build a simple dialog
based app which performs FTP uploads and downloads to any FTP server.
Copyright
- You are allowed to include the source code in any product (commercial, shareware,
freeware or otherwise) when your product is released in binary form.
- You are allowed to modify the source code in any way you want except you
cannot modify the copyright details at the top of each module.
- If you want to distribute source code with your application, then you are
only allowed to distribute versions released by the author. This is to maintain
a single distribution point for the source code.
Updates
v1.24 (17 April 2022)
- Updated copyright details.
- Updated the code to use C++ uniform initialization for all variable
declarations.
v1.23 (12 December 2021)
- Updated copyright details.
- Fixed more Clang-Tidy static code analysis warnings in the code.
v1.22 (4 April 2020)
- Updated copyright details.
- Fixed more Clang-Tidy static code analysis warnings in the code.
v1.21 (27 December 2019)
- Fixed various Clang-Tidy static code analysis warnings in the code.
v1.20 (29 September 2019)
- Fixed a number of compiler warnings when the code is compiled with VS
2019 Preview
v1.19 (6 May 2019)
- Updated copyright details.
- Updated the code to clean compile on VC 2019
v1.18 (6 December 2018)
- Updated copyright details.
- Fixed a number of C++ core guidelines compiler warnings. These changes
mean that the code will now only compile on VC 2017 or later.
- Changed enums to enum class throughout codebase
- Reworked the CFTPTransferer class to be independent of MFC
- Reworked all the notifications from the worker thread in CFTPTransferDlg
to the UI to use PostMessage
v1.17 (23 December 2017)
- Fix compile problems in CFTPTransferer::CreateSession in VC2017
- Updated the code to compile cleanly when _ATL_NO_AUTOMATIC_NAMESPACE is
defined.
v1.16 (5 October 2017)
- Replaced CString::operator LPC*STR() calls throughout the codebase with
CString::GetString calls.
v1.15 (24 January 2017)
- Updated copyright details
- Updated the code to clean compile on VC 2010 to 2015
- Replaced BOOL with bool throughout the codebase
- Updated the code to clean compile using /analyze
- Replaced NULL with nullptr throughout the codebase. This means that the
code now requires VC 2010 at a minimum to compile.
- Implemented support for send and receive timeouts
v1.14 (18 May 2008)
- Updated copyright details
- Addition of AttachSession and DetachSession methods which allow the lifetime
of the session to be controlled independently of the lifetime of the CFTPTransferer
instance. Thanks to Hans Dietrich for prompting this update
- Updated the logic in CFTPTransferDlg::OnWininetStatusCallBack to correctly
handle ASCII or Unicode strings. Thanks to Hans Dietrich for prompting this
update.
- Fixed a spelling mistake in the IDS_FTPTRANSFER_RETRIEVING_FILE string resource.
v1.13 (30 December 2007)
- Updated the code to remove VC 6 style appwizard comments.
- Updated the sample apps to clean compile on VC 2008.
- CFTPTransferer::GetErrorMessage now uses the FORMAT_MESSAGE_IGNORE_INSERTS
flag. For more information please see Raymond Chen's blog at
http://blogs.msdn.com/oldnewthing/archive/2007/11/28/6564257.aspx. Thanks
to Alexey Kuznetsov for reporting this issue.
- CFTPTransferer::GetErrorMessage now uses Checked::tcsncpy_s if compiled
using VC 2005 or later.
v1.12 (10 June 2007)
- Minor bug fix to CFTPTransferDlg::SetTimeLeft to correctly handle 64 bit
integer parameter.
v1.11 (18 May 2007)
- Updated documentation to say that resume is not supported.
- Updated documentation to better describe how to add the classes into your
application. Thanks to Roger Huggins for prompting this update.
v1.10 (14 April 2007)
v1.09 (23 November 2006)
- Updated the code to clean compile on VC 2005
- Updated copyright details
- Updated the code to compile correctly for Win64
- Integrated the _FTP_DOWNLOAD_DATA class into the CFTPTransferDlg class
- Optimized member variable construction in CFTPTransferDlg constructor
- Code now uses newer C++ style casts in preference to C style casts
- Refactored the code to produce a new CFTPTransferer class which provides
a synchronous interface to Wininet FTP which CFTPTransferDlg now inherits from.
This is in line with the most recent updates in the author's CHttpDownloadDlg
class
- Class now handles extra large files (> 4GB)
- Code now correctly handles ERROR_INTERNET_EXTENDED_ERROR errors
- Updated documentation to use same style as the web site.
v1.08 (13 June 2003)
- Now supports connection timeouts since wininet doesn't <ggg>.
- Other various tidy ups to the code, such as review of all the TRACE statements
and calls to GetLastError
v1.07 (30 October 2002)
- Fixed a problem with seeking to the correct position when resuming a download.
Thanks to Liping Dai for this bug report.
5 June 2002
- Renamed some string resources used by the class to maintain naming consistency.
v1.06 (20 April 2002)
- Modified the code to correctly support FTP resumes. Feeedback from jony
for reporting this issue.
- Fixed a bug in the call to AfxBeginThread.
v1.05 (2 February 2002)
- Now includes support for FTP resumes via the FTP command "REST". Please
note that IE 5.01 SP2 is required to support this. This support is experimental,
so your feedback is welcome.
- Updated documentation to include explicit requirements for the class.
v1.04 (17 October 2001)
- Now includes support for PASV
- Fixed a Unicode issue in CFTPTransferDlg::OnStatusCallBack
- Support for precongfigured or direct settings.
- Support for proxies
v1.03 (10 October 2001)
- Fixed a problem where old style MFC exception handling was being used instead
of C++ standard.
v1.02 (11 June 2001)
- Updated copyright details.
- Now provides Bandwidth throtling support. Thanks to Karim Mribti for this
good addition.
- Minor code tidy up thanks to BoundsChecker
- m_bPromptForOverwrite now works correctly for downloads as well as uploads
- Fixed a problem with the progress control not updating correctly
10 December 2000
- Updated distribution to describe when you need to "#include" and
link to.
v1.01 (31 October 2000)
- Added a m_bPromptOverwrite variable
- Fixed a bug in the way the progress control was being updated.
v1.0 (4 January 2000)