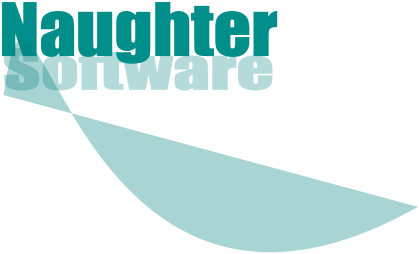
The first class implements an MFC CDialog derived class which performs HTTP downloads
similar to the old Internet Explorer download dialog as shown below:
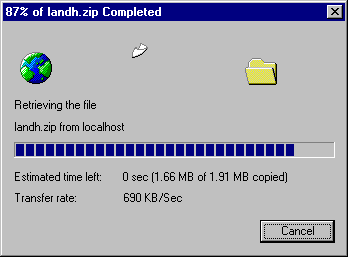
To use CHTTPDownloadDlg in your project simply include HttpDownloadDlg.cpp/h
from the test application in your application and #include "HttpDownloadDlg.h"
in whichever files you want to use the class in. To bring up the dialog to download
a specific file, just use some code like the following:
CHTTPDownloadDlg dlg;
dlg.m_sURLToDownload = _T("http://www.some-site.com/somefile.ext");
dlg.m_sFileToDownloadInto = _T("c:\\somefile.ext");
if (dlg.DoModal()
== IDOK)
AfxMessageBox(_T("File was downloaded successfully"));
The CHTTPDownloader class is used internally by CHTTPDownloadDlg and allows you
to operate in a synchronous non-UI manner as follows:
CHTTPDownloader downloader;
downloader.m_Settings.m_sURLToDownload = _T("http://www.some-site.com/somefile.ext");
downloader.m_Settings.m_sFileToDownloadInto = _T("c:\\somefile.ext");
downloader.Download();
Finally if you want to use the WinHTTP stack instead of Wininet, then you can
use CHTTPDownloadDlg2 and CHTTPDownloader2 instead of CHTTPDownloadDlg and CHTTPDownloader.
The enclosed zip file contains the
CHTTPDownloadDlg, CHTTPDownloader, CHTTPDownloadDlg2 & CHTTPDownloader2 source
code and 2 simple MFC dialog based programs which exercises the classes.
Copyright
- You are allowed to include the source code in any product (commercial, shareware,
freeware or otherwise) when your product is released in binary form.
- You are allowed to modify the source code in any way you want except you
cannot modify the copyright details at the top of each module.
- If you want to distribute source code with your application, then you are
only allowed to distribute versions released by the author. This is to maintain
a single distribution point for the source code.
Updates
v1.42 (30 April 2022)
- Updated copyright details.
- Updated the code to use C++ uniform initialization for all variable
declarations.
v1.41 (16 January 2021)
- Updated copyright details.
- Updated the code to use std::atomic for thread synchronisation.
- Replaced ATL::CHeapPtr with std::vector.
- General cleanup /
review of the code
v1.40 (12 January 2020)
- Updated copyright details.
- Fixed more Clang-Tidy static code analysis warnings in the code.
v1.39 (8 December 2019)
- Fixed a bug in CHTTPDownloader::Download and CHTTPDownloader2::Download
where an unsuccessfully downloaded file would not be deleted. Thanks to
"michar" for reporting this bug.
- Fixed various Clang-Tidy static code analysis warnings in the code.
v1.38 (12 October 2019)
- Fixed a number of compiler warnings when the code is compiled with VS
2019 Preview
- Reworked timing code to use GetTickCount64
v1.37 (30 April 2019)
- Updated copyright details.
- Updated the code to clean compile on VC 2019
v1.36 (27 October 2018)
- Updated copyright details.
- Fixed a number of C++ core guidelines compiler warnings. These changes
mean that the code will now only compile on VC 2017 or later.
v1.35 (28 December 2017)
- Updated the code to compile cleanly when _ATL_NO_AUTOMATIC_NAMESPACE is
defined.
v1.34 (19 September 2017)
- Updated copyright details.
- Replaced CString::operator LPC*STR() calls throughout the codebase with
CString::GetString calls.
- Fixed compiler errors in CHTTPDownloader::CreateSession.
- Fixed compiler errors in CHTTPDownloader2::CreateSession.
v1.33 (25 December 2016)
- Updated code to use nullptr instead of NULL
- Updated usage of BOOL with bool in codebase
- Reworked CHTTPDownloader::Abort to use proper thread protection
- Reworked CHTTPDownloader2::Abort to use proper thread protection
- Removed support for the m_bIgnoreInvalidCertificate & m_bIgnoreCRLFailures
configuration values and introduced support for SECURITY_FLAG_IGNORE_REVOCATION,
SECURITY_FLAG_IGNORE_UNKNOWN_CA, SECURITY_FLAG_IGNORE_WRONG_USAGE, SECURITY_FLAG_IGNORE_CERT_CN_INVALID,
SECURITY_FLAG_IGNORE_CERT_DATE_INVALID, SECURITY_FLAG_IGNORE_REDIRECT_TO_HTTPS &
SECURITY_FLAG_IGNORE_REDIRECT_TO_HTTP option flags in the CHTTPDownloader class.
- Removed support for the m_bIgnoreInvalidCertificate configuration value
and introduced support for SECURITY_FLAG_IGNORE_UNKNOWN_CA, SECURITY_FLAG_IGNORE_WRONG_USAGE,
SECURITY_FLAG_IGNORE_CERT_CN_INVALID & SECURITY_FLAG_IGNORE_CERT_DATE_INVALID
option flags in the CHTTPDownloader2 class
v1.32 (16 January 2016)
- Updated copyright details.
- Verified the code compiles cleanly in VC 2015
- Added SAL annotations to all the code
v1.31 (22 February 2015)
- Updated copyright details.
- Updated the sample project settings to more modern default values
- Renamed CHttpDownloader class to CHTTPDownloader
- Renamed CHttpDownloadDlg class to CHTTPDownloadDlg
- Renamed CHttpDownloader2 class to CHTTPDownloader2
- Renamed CHttpDownloadDlg2 class to CHTTPDownloadDlg2
- Updated the code to clean compile using /analyze
- Added support for a CHTTPDownloader::m_bIgnoreInvalidCertificate value.
Setting this value to TRUE, the code will ignore the ERROR_INTERNET_INVALID_CA
error code when downloading (i.e. an invalid HTTPS certificate)
- Added support for a CHTTPDownloader::m_bIgnoreCRLFailures. Setting this
value to TRUE, the code will ignore the ERROR_INTERNET_SEC_CERT_REV_FAILED error
code when downloading (i.e. revocation checks failed)
- Added support for a CHTTPDownloader2::m_bIgnoreInvalidCertificate value.
Setting this value to TRUE, the code will ignore an errors related to invalid
HTTPS certificates
6 January 2014
- Minor update to the sample code snippet on this web page. Thanks to David
Crow for reporting this issue.
v1.30 (08 June 2008)
- Updated code to compile correctly using _ATL_CSTRING_EXPLICIT_CONSTRUCTORS
define
- Code now compiles cleanly using Code Analysis (/analyze)
- The code has now been updated to support VC 2005 or later only.
v1.29 (11 May 2008)
- Updated the logic in CHttpDownloadDlg::OnWininetStatusCallBack to correctly
handle ASCII or Unicode strings. Thanks to Hans Dietrich for prompting this
update.
- Updated copyright details
- Addition of AttachSession and DetachSession methods which allow the lifetime
of the session to be controlled independently of the lifetime of the CHttpDownloader(2)
instance. Thanks to Hans Dietrich for prompting this update
- Fixed a spelling mistake in the IDS_HTTPDOWNLOAD_RETRIEVING_FILE string
resource
- OnProgress virtual methods now correctly handles reporting results for resumed
downloads
v1.28 (30 December 2007)
- Updated sample apps to clean compile on VC 2008
- Remove VC 6 style appwizard comments from the code.
- CHttpDownloader::GetErrorMessage now uses the FORMAT_MESSAGE_IGNORE_INSERTS
flag. For more information please see Raymond Chen's blog at
http://blogs.msdn.com/oldnewthing/archive/2007/11/28/6564257.aspx. Thanks
to Alexey Kuznetsov for reporting this issue.
- CHttpDownloader::GetErrorMessage now uses Checked::tcsncpy_s if compiled
using VC 2005 or later.
- CHttpDownloader2::GetErrorMessage now uses the FORMAT_MESSAGE_IGNORE_INSERTS
flag. For more information please see Raymond Chen's blog at
http://blogs.msdn.com/oldnewthing/archive/2007/11/28/6564257.aspx. Thanks
to Alexey Kuznetsov for reporting this issue.
- CHttpDownloader2::GetErrorMessage now uses Checked::tcsncpy_s if compiled
using VC 2005 or later.
v1.27 (10 June 2007)
- Updated comments in documentation about usage of the Platform SDK.
- Updated copyright details.
- Updated CHttpDownloadDlg::OnWininetStatusCallBack in line with CFTPTransferer
class.
- CHttpDownloader now supports resumed downloads for files > 4GB in size.
- CHttpDownloader2 now supports resumed downloads for files > 4GB in size.
- Updated sample app to compile cleanly on VC 2005
v1.26 (17 September 2006)
- Fixed an issue where the OnStatusCallback method was not being called. This
has been addressed by renaming the function to CHttpDownloadDlg2::OnWinHttpStatusCallBack.
This is necessary due to the refactoring of the base CHttpDownloader2 class.
Thanks to Johan S�rensen for reporting this bug
- Fixed an issue where the OnStatusCallback method was not being called. This
has been addressed by renaming the function to CHttpDownloadDlg::OnWininetStatusCallBack.
This is necessary due to the refactoring of the base CHttpDownloader class.
Thanks to Johan S�rensen for reporting this bug
v1.25 (25 August 2006)
- Downloading to file is now optional for both CHttpDownloader and CHttpDownloader2,
a new virtual function OnDownloadData is now called each time a chunk of data
has been retrieved. The default implementation writes the data into the file "m_sFileToDownloadInto".
A derived class is free to override this function and implement their own specific
functionality. Thanks to Itamar Syn-Hershko for suggesting this update.
- Optimized the way aborting a download occurs by freeing up the handles in
the SetAbort call. This should hopefully fix times where aborting a download
appears to respond slowly.
- Class now supports sending client certificates via user selection and programmatically.
Thanks to Johan S�rensen for this very nice addition.
v1.24 (16 July 2006)
- Split off CHttpDownloader class into its own module of HttpDownloader.cpp/h.
- Implemented a WinHTTP version of CHttpDownloader called CHttpDownloader2.
- Implemented a WinHTTP version of CHttpDownloadDlg called CHttpDownloadDlg2.
- Now includes a similar sample app in the download to demonstrate the WinHTTP
functionality.
- Now includes support for customizing the HTTP_REFERRER header
- Now includes support for customizing the HTTP verb used e.g. "GET"
etc
- Now includes support for specifying the HTTP version to use e.g. "HTTP/1.0". "HTTP/1.1"
etc
- Now includes support for customizing the HTTP_ACCEPT header
- Added support for client customization of any other HTTP headers which are
now transmitted
- Brought the CHttpDownloadSettings member variables back into the CHttpDownloader
class. This means that the HttpDownloadSettings module is now defunct.
- In line with the new CHttpDownloader2 class, the creation of the various
handles in now broken down into 3 virtual functions of CreateSession, CreateConnection
and CreateRequest.
- Now supports both HTTP and Proxy preauthentication.
- Reviewed all TRACE statements for correctness
- internet handles are now freed up before CHttpDownloader::Download returns
- Moved the m_lAbort flag from the CHttpDownloadDlg up into CHttpDownloader.
This allows client code extra flexibility in how users can cancel a download.
Thanks to Itamar Syn-Hershko for reporting this issue.
v1.23 (15 July 2006)
- Fixed some issues in OnStatusCallback when the code is compiled for Unicode.
Thanks to Itamar Syn-Hershko for reporting this issue.
- WM_HTTPDOWNLOAD_THREAD_FINISHED now uses WM_USER instead of WM_APP to define
its value
- Major refactoring of the code into 3 classes namely CHttpDownloadSettings,
CHttpDownloader and the existing CHttpDownloadDlg class which has been significantly
reworked to fit in with this new layout. The new layout means that you can use
the new "CHttpDownloader" class in a synchronous manner without any
UI at all. For example to download a file without any UI you would just use
something like:
CHttpDownloader downloader;
downloader.m_Settings.m_sURLToDownload
= _T("http://www.some-site.com/somefile.ext");
downloader.m_Settings.m_sFileToDownloadInto
= _T("c:\\somefile.ext");
downloader.Download();
When the
Download() function returns the file will have been downloaded successfully
or will have failed to download. Various synchronous virtual functions can be
overridden to handle the various events as they occur during the download. The
CHttpDownloadDlg class
now derives from both CDialog and CHttpDownloader,
and handles these virtual events to provide the existing functionality which
it had. You should review how CHttpDownloadDlg handles these virtual functions
if you want to create your own customized implementation which derives from
CHttpDownloader.
- Fixed a spelling mistake in the IDS_HTTPDOWNLOAD_RETREIVEING_FILE string
- The buffer size used in calls to InternetReadFile can now be changed via
CHttpDownloadSettings::m_dwReadBufferSize
- Thread protected changes to the Abort flag
v1.22 (2 July 2006)
- Updated the code to clean compile on VC 2005
- Code now uses new C++ style casts rather than old style C casts where necessary.
- The class now requires the Platform SDK if compiled using VC 6.
v1.21 (8 May 2006)
- Updated copyright details.
- Fixed an ASSERT in CHttpDownloadDlg::OnThreadFinished when you cancel a
download prematurely.
- Updated the documentation to use the same style as the web site.
- Did a spell check of the documentation.
- Addition of a m_bNoURLRedirect variable so that the class can optionally
ignore 302 redirects.
- The flags passed to HttpOpenRequest can now be customized via a new method
GetOpenRequestFlags
- Optimized creation of member variables in CHttpDownloadDlg constructor.
- Updated various captions and version infos in the sample app
- Fixed a number of compiler warnings when the code is compiled using /Wp64.
Please note that to implement these fixes, the code will now require the Platform
SDK to be installed if you compile the code on VC 6.
v1.20 (4 April 2005)
- Fix for bug when providing all information in the dialog for both proxy
and user validation and one uses the option to connect directly via the proxy
and no prompting is checked then when the code 407 is received the code resends
with proxy info and gets a 401 code. This time it reports the error as the bool
flag for bRetrying is true. Thanks to Stephen Miller for providing the fix for
this problem.
v1.19 (5 March 2005)
- Tidied up the way the file to be downloaded is closed and deleted if the
download fails. Thanks to Guillermo for reporting this bug.
- Fixed a bug in the main logic of the class which caused the http or proxy
credentials dialog to be continually displayed even when you hit cancel. Thanks
to Guillermo for reporting this bug and providing the fix.
- Optimized the code which calls GetLastError.
- Display of error messages now uses FormatMessage to display a more friendlier
value.
v1.18 (11 November 2004)
- Fixed an issue in the code where the request is only reissued when authentication
errors occur and the code needs to prompt the user. Thanks to Thomas Kiesswetter
for reporting this problem and provided the fix.
- Addition of a CHTTPDOWNLOADDLG_EXT_CLASS preprocessor macro which allows
the class to be easily added to an extension dll
- Fixed a bug when errors are displayed when QueryStatusCode fails.
v1.17 (12 February 2004)
- Class now automatically pulls in wininet.lib support via a #pragma. This
means that client apps no longer need to update the linker settings.
v1.16 (5 February 2004)
- Updated the way the progress control is displayed when a resumed download
is performed. Thanks to David C. Hochrein for reporting this addition.
v1.15 (29 June 2003)
- The progress control now uses a range of 0 - 100 percent rather than the
actual file size. This prevents overflow with the SetRange method. I could use
SetRange32, but then the class would depend on having an updated version of
the common control dll installed.
- Fixed a problem in OnStatusCallBack when compiled for Unicode.
v1.14 (19 January 2003)
- Fixed a bug in calling InternetReadFile when doing proxy authentication
which could have resulted in an infinite loop occurring. Thanks to Nathan Lewis
for reporting this.
- Reworked the code which queries for the HTTP status code to use the HTTP_QUERY_FLAG_NUMBER
flag in the call to HttpQueryInfo. Again thanks to Nathan Lewis for suggesting
this.
- Reworked the code which queries for the HTTP content length code to use
the HTTP_QUERY_FLAG_NUMBER flag in the call to HttpQueryInfo. Again thanks to
Nathan Lewis for suggesting this.
v1.13 (6 January 2003)
- Fixed a bug where using HTTPS on a non standard port would cause the download
to fail.Thanks to "Sundar" for spotting this problem
- Fixed a bug in the displaying of errors which use the strings "IDS_HTTPDOWNLOAD_INVALID_SERVER_RESPONSE"
and "IDS_HTTPDOWNLOAD_INVALID_SERVER_RESPONSE"
v1.12 (30 October 2002)
- Fixed a problem with seeking to the correct position when resuming a download.
Thanks to Liping Dai for this bug report.
v1.11 (20 April 2002)
- Fixed bug in call to AfxBeginThread. Thanks to mark anderson for spotting
this problem.
- Fixed a redraw glitch where the progress control was being updated twice
instead of once each time for each percentage. Again thanks to Mark Anderson
for spotting this problem.
v1.10 (2 February 2002)
- Fixed a bug where the local downloaded file would get appended to even when
you are not resuming the download. Thanks to Davide Zaccanti for spotting this
problem.
- Updated documentation to include explicit requirements for the class.
v1.09 (3 December 2001)
- Tidied up support for resumed downloads.
v1.08 (10 October 2001)
- Fixed a problem where old style MFC exception handling was being used instead
of C++ standard.
- Code is now independent of Platform SDK meaning that it can compile on VC
5 without need for having the Platform SDK installed.
v1.07 (17 June 2001)
- Now provides Bandwidth throtling support. Thanks to Karim Mribti for this
good addition.
- Code now uses InternetReadFile instead of InternetReadFileEx as InternetReadFileEx
is not implemented under Unicode.
v1.06 (28 April 2001)
- Updated Copyright Information
- You need to have the Latest Wininet header files installed to compile the
code. This will not be the case with VC 5, which you will also have to install
the plaform SDK also.
- Now includes support for the following fields:
m_sProxyUserName: You
can now specify the Proxy user details
m_sProxyPassword: THe Proxy password
to use
m_ConnectionType: You can now specify the HTTP connection type
m_bPromptFileOverwrite: Should you be prompted for file overwrites
m_bPromptForProxyDetails:
Should you be prompted for Proxy authentication
m_bPromptForHTTPDetails:
Should you be prompted for HTTP authentication
m_sUserAgent: The User Agent
value to use in HTTP requests
- Fixed a problem in downloading files using HTTPS.
- Provided a OnSetOptions method to allow customisation through deriving your
own class from CHttpDownloadDlg.
v1.05 (2 July 2000)
- Now supports resuming downloads
v1.04 (12 June 2000)
- Now fully supports HTTPS downloads.
v1.03 (25 January 2000)
- Fixed a problem where server authentication was not being detected correctly,
while proxy authentication was being handled.
- Updated the way and periodicity certain UI controls are updated during the
HTTP download.
v1.02 (29 November 1999)
- Fixed a number of compiler warnings and errors when used in VC++ 6.0.
v1.01 (15 November 1999)
- Updated documentation to refer to what rc resources are needed by the class.
- Renamed all resources used from "ASYNC_DOWLOAD" to include "HTTPDOWNLOAD".
- Now handles HTTP error 407 (Proxy Authentication) and HTTP error 401 (Server
Authentication). You are prompted with a password dialog when either of these
forms of authentication are required.
- Sample program now allows you to specify the URL to download from aswell
as the file to download into and the username and password to connect with.
- The download file is now deleted if the download was aborted or if some
other error occurs during the download.
- Parsing of URL will now work where "http://" is not explicitely
specified.
- Improved the general look and feel of the demo app and called it "HTML
File Downloader".
v1.0 (14 November 1999)