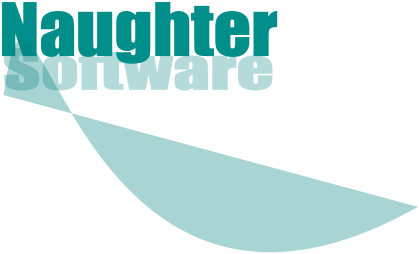
JSON++ v1.15 A set of C++ classes to support
JSON / JSON5
I have been working with JSON recently in a number of C++ projects and wanted
to learn it by understanding the JSON specification by writing my own JSON parser
/ encoder. There are a number of nice JSON libraries for C++ out there such as
SimpleJSON and
JSON for Modern C++. I particularly
liked the SimpleJSON library which was able to implement a nice JSON parser / encoder
in c. 1500 lines of code. I decided to write my own class based on SimpleJSON but
using some modern C++ features such as r-value references, move constructors and
operator=. Thus was born JSON++. Included in the download is the JSON++ source code
and a VC 2017 application to fully exercise all the functionality of JSON++. The
main class provided is CValue and is implemented in a JSONPP namespace. All the
constructors of the CValue class allow a JSON value object to be constructed directly
from other C++ types such as bool, double, long, STL strings, STL vectors, STL maps
etc. Standard C++ operator= methods are also provided which mirror the constructor
methods. The class also supports construction and assignment from C++ r-value references.
A "Parse" method of CValue allows a JSON encoded string (either UTF8 or
UTF16) to be parsed into the CValue instance. An "Encode" method allows
a JSON encoded string in the form of a std::wstring to be created from a CValue
instance. Various other CValue methods are modeled on the SimpleJSON classes.
Features
- Provides a complete JSON and JSON5 parser and encoder in c. 2300 lines of C++.
- All the code is provided in a single C++ Header only module of "JSON++.h"
.
- All the code clean compiles at warning level 4, is /analyze and /permissive-
clean and includes ASCII, Unicode, x86 and x64 built configurations.
- The class supports both UTF8 and UTF16 encoded JSON.
- Performance testing of the code has shown that JSON++ is about 50% faster
than SimpleJSON for parsing. This is mainly achieved through the use of r-value
references and placement new to avoid use of explicit heap memory allocations
which SimpleJSON is forced to use. The encoding speed of JSON++ is the same
as SimpleJSON, although support is provided in JSON++ for pretty printing the
resulting JSON.
- Performance testing for parsing of a c. 400 character UTF16 JSON string
on my Core I7 quad core 4Ghz development machine indicates a performance of
about 30 MB / second.
- Encoding performance works out at about 100,000 encodes per second for a
c. 400 character JSON string on my Core I7 machine.
- If a parsing error occurs during a call to the CValue::Parse method, then
a JSONPP::CParseException will be thrown. This class provides a "m_Reason"
enum value which specifies why the parsing failed and a "m_nIndex"
value which specifies where the parsing error occurred in the JSON encoded text.
- The classes are only supported on VC 2017 or later.
- Provides support for JSON pointer (https://tools.ietf.org/html/rfc6901)
via a simple JSONPP::CValue::JSONPointer method.
- Provides support for JSON5 (https://spec.json5.org/)
parsing and encoding in addition to JSON.
The enclosed zip file contains the JSON++ source
code and a VC 2017 console based application which exercises all of the JSON++ functionality.
Copyright
- You are allowed to include the source code in any product (commercial, shareware,
freeware or otherwise) when your product is released in binary form.
- You are allowed to modify the source code in any way you want except you
cannot modify the copyright details at the top of each module.
- If you want to distribute source code with your application, then you are
only allowed to distribute versions released by the author. This is to maintain
a single distribution point for the source code.
Usage
- To use the JSON++ classes in your project simply #include "JSON++.h"
in which ever of the modules in your application requires the JSON functionality.
The header file will look after pulling in any dependent header files
- As the code internally uses std::variant, your project will need to
specify the /std:c++17 compiler setting or later.
- That should be it. You should now have a fully functional JSON parser and
encoder now available to your Windows C++ application.
Updates
v1.15 (9 July 2023)
- Updated the class to provide support for JSON5 (https://spec.json5.org/)
parsing and encoding.The JSON5 implementation in JSON++ follows the
guidelines which SQLite3 used (https://www.sqlite.org/json1.html#json5)
when it was updated to support JSON5. To support parsing of JSON5, you can
pass the JSONPP::PARSE_FLAGS::PARSE_JSON5 value as the third parameter to
the CValue::Parse method. If you pass a zero instead for this parameter then
the method will revert to strict JSON parsing. To support JSON5 encoding you
can pass the JSONPP::ENCODE_FLAGS::ENCODE_JSON5 value as the first parameter
to the CValue::Encode method. Please see the example code in main.cpp for
further examples.
v1.14 (12 May 2023)
- Updated copyright details
- Updated module to indicate that it needs to be compiled using
/std:c++17. Thanks to Martin Richter for reporting this issue.
v1.13 (22 April 2022)
- Fixed a bug in CValue::operator=(const wchar_t*) where the m_Data member
variable would get initialized incorrectly to a bool on VS 2022. Thanks to
"jls" for reporting this issue.
v1.12 (29 January 2022)
- Updated copyright details.
- Fixed more static code analysis warnings in Visual Studio 2022.
- Updated the code to use C++ uniform initialization for all variable
declarations.
v1.11 (2 November 2021)
- Updated copyright details.
- Updated compiler settings to more modern defaults.
v1.10 (23 May 2020)
- Added const versions of the CValue::Child methods.
- Added [[nodiscard]] to a number of CValue methods.
v1.09 (29 January 2020)
- Updated copyright details.
- Fixed more Clang-Tidy static code analysis warnings in the code.
v1.08 (16 December 2019)
- Fixed various Clang-Tidy static code analysis warnings in the code.
v1.07 (3 August 2019)
- Added comprehensive SAL annotations to all the code.
v1.06 (22 April 2019)
- Updated copyright details.
- Updated the code to clean compile on VC 2019.
v1.05 (2 September 2018)
- Fixed a number of compiler warnings when using VS 2017 15.8.2.
v1.04 (31 July 2018)
- Reworked code to use Windows API calls MultiByteToWideChar API for
the UTF8 to UTF16 conversion rather than the deprecated
codecvt header.
v1.03 (30 June 2018)
- Reworked the implementation to use std::variant. This does mean that the
code now requires at least the /std:c++17 compiler setting. This has
resulted in the module shrinking by c. 160 lines. Testing shows the
performance remains the same as before.
v1.02 (29 May 2018)
- Fixed a number of C++ core guidelines compiler warnings.
v1.01 (10 January 2018)
- Updated copyright details.
- Added support for JSON Pointer (https://tools.ietf.org/html/rfc6901)
support to the code via a new CValue::JSONPointer method.
- Made a number of methods of the CValue class static as they did not need
to be non-static.
V1.0 (25 November 2017)