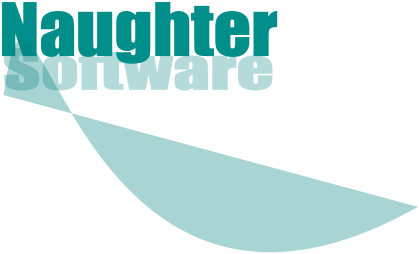
Welcome to SQLiteWrappers, a set of C++ classes to encapsulate SQLite v3. The
code below shows a simple example to open a database and add a row to a table called "tblTest"
with one text column (without any error handling!):
SQLite3:CDB db;
db.Open16(L"database.db");
db.Exec("INSERT
INTO tblTest VALUES ('sample text');");
Here's a further example to run a simple parameterized query to do an INSERT
on the previously mentioned "tblTest":
SQLite3::CStatment stmt;
stmt.Prepare16v2(db, L"INSERT INTO tblTest VALUES(?);");
char* szData = "More Sample Text";
stmt.Bind(1, szData);
stmt.Step();
The classes provided are as follows:
- CDB which represents a database, or in other words sqlite3* pointer.
- CValue which represents a value stored in a database, or in other
words sqlite3_value* pointer.
- CStatement which represents a statement, or in other words a sqlite3_stmt*
pointer.
- CValue2 which represents a combined CValue and CStatement (useful
when you want to have a CValue instance with the same lifetime as a CStatement).
- CBlob which represents a blob in SQLite or in other words a sqlite3_blob*
pointer.
- CPtr which encapsulates something which needs to be freed using sqlite3_free
as well as printf style formatting of SQL Strings using sqlite3_vmprintf.
- CRowset provides an STL compliant encapsulation of a rowset as returned
from SQLite3::CStatment::ExecRowset[16].
- CRowset2 which represents a combined CRowset and CStatement (useful
when you want to have a CRowset instance with the same lifetime as a CStatement
e.g. SQLite3::CDB::ExecRowset[16]).
- CRowset::iterator which is of type SQLite3::CRowsetIterator which
provides an STL compliant input iterator to iterate across the contents of SQLite3::CRowset.
- CRow represents an individual row of a rowset as provided by SQLite3::CRowset::iterator.
- CRow::iterator which provides a STL random access iterator to iterate
across the columns of a CRow.
Features
- A complete wrapper for SQLite v3.50.1 functionality.
- Hides the complexity of sqlite3 printf functionality.
- Automatic and optional resource de-allocation of all SQLite resource types
in C++ destructors
- Classes have no external dependencies on other libraries such as ATL or
MFC.
- Provides a thin veneer over the SQLite3 C API so you should be able to easily
map your existing C style SQLite code to C++ without many changes. All errors
are still indicated using return values from methods as opposed to using exceptions.
Another reason this approach makes sense is that it is difficult to distinguish
between some return values from SQLite, e.g. not all return values from sqlite3_step
are errors but indicate conditions which client code may need to react to e.g.
SQLITE_ROW or SQLITE_DONE.
- Provides STL iterator access to the returned rowset. The class returned
from CStatement::ExecRowset is SQLite3::CRowset which provides an input STL
iterator to traverse though all the rows returned by SQLite3::CStatement::Step.
Each row as returned from the CRowset iterator is represented by a SQLite3::CRow.
Finally SQLite3::CRow provides a random access STL iterator to all the columns
returning a SQLite3::CValue. This allows you to write code which iterates across
all rows and columns as follows:
SQLite3::CRowset2 rowset;
db.ExecRowset16(L"SELECT * FROM tblTest", rowset);
for (auto row : rowset)
{
for (auto column : row)
printf("%s ",
column.Text());
printf("\n");
}
- Provides validation of SQLite resource pointers in all method calls.
- Provides ExecScalar[16] methods in the CDB class modelled on the same method
name as provided by the CppSQLite C++ classes on CodeProject at
http://www.codeproject.com/Articles/6343/CppSQLite-C-Wrapper-for-SQLite.
Unlike the code there, my version of ExecScalar will handle any data type through
the use of the CValue2 class. This allows you to write the following (without
any error handling!) which returns the number of rows (as a 64 bit quantity)
in a table called "tblTest":
SQLite3::CValue2 rowCount;
db.Exec16Scalar(L"SELECT COUNT(*) FROM tblTest", rowCount);
printf("The
number of rows in tblTest is %I64d\n", rowCount.Int64());
The enclosed zip file contains the
SQLiteWrappers source code and a simple VC 2017 console based application which
demonstrates most of the classes functionality.
Copyright
- You are allowed to include the source code in any product (commercial, shareware,
freeware or otherwise) when your product is released in binary form.
- You are allowed to modify the source code in any way you want except you
cannot modify the copyright details at the top of each module.
- If you want to distribute source code with your application, then you are
only allowed to distribute versions released by the author. This is to maintain
a single distribution point for the source code.
Updates
v1.51 (15 June 2025)
- Verified the code works against SQLite v3.50.1
v1.50 (1 June 2025)
- Updated the code to compile against SQLite v3.50.0. Added support for
sqlite3_setlk_timeout.
v1.49 (4 March 2025)
- Verified the code works against SQLite v3.49.1
v1.48 (15 February 2025)
- Verified the code works against SQLite v3.49.0
v1.47 (22 January 2025)
- Updated copyright details.
- Verified the code works against SQLite v3.48.0
v1.46 (15 December 2024)
- Verified the code works against SQLite v3.47.2
v1.45 (29 November 2024)
- Verified the code works against SQLite v3.47.1
v1.44 (27 October 2024)
- Verified the code works against SQLite v3.47.0
v1.43 (24 August 2024)
- Verified the code works against SQLite v3.46.1
v1.42 (1 June 2024)
- Verified the code works against SQLite v3.46.0
v1.41 (21 April 2024)
- Verified the code works against SQLite v3.45.3
v1.40 (28 March 2024)
- Verified the code works against SQLite v3.45.2
v1.39 (5 February 2024)
- Verified the code works against SQLite v3.45.1
v1.37 (12 January 2024)
- Updated copyright details.
- Verified the code works against SQLite v3.44.2
v1.36 (2 November 2023)
- Updated the code to compile against SQLite v3.44.0. Added support for
sqlite3_get_clientdata, sqlite3_set_clientdata & sqlite3changeset_upgrade.
17 September 2023
- Verified the code works against SQLite v3.43.1.
v1.35 (27 August 2023)
- Updated the code to compile against SQLite v3.43.0. Added support for
sqlite3_stmt_explain.
11 June 2023
- Verified the code works against SQLite v3.42.0.
19 March 2023
- Verified the code works against SQLite v3.41.1.
v1.34 (24 February 2023)
- Updated copyright details.
- Updated the code to compile against SQLite v3.41.0. Added support for
sqlite3_is_interrupted & sqlite3_stmt_scanstatus_v2.
29 December 2022
- Verified the code works against SQLite v3.40.1.
v1.33 (23 November 2022)
- Updated the code to compile against SQLite v3.40.0. Added support for
sqlite3_filename typedef & sqlite3_value_encoding.
8 October 2022
- Verified the code works against SQLite v3.39.4.
18 September 2022
- Verified the code works against SQLite v3.39.3.
6 August 2022
- Verified the code works against SQLite v3.39.2.
v1.32 (16 July 2022)
- Updated the code to compile against SQLite v3.39.1. Added support for
sqlite3_db_name.
v1.31 (18 March 2022)
- Updated the code to compile against SQLite v3.38.
- Added support for
sqlite3_error_offset, sqlite3_vtab_in_first & sqlite3_vtab_in_next.
v1.30 (12 February 2022)
- Updated the code to use C++ uniform initialization for all variable
declarations
v1.29 (21 January 2022)
- Updated copyright details.
- Updated the code to compile against SQLite v3.37.2.
Added support for sqlite3_int64 sqlite3_changes64, sqlite3_total_changes64 &
sqlite3_autovacuum_pages.
- Fixed more Clang-Tidy static code analysis warnings in the code.
- Fixed compiler warnings when compiled using Visual Studio 2022.
v1.28 (11 July 2021)
- Updated the code to compile against SQLite v3.36.0. Added support for
sqlite3_preupdate_hook, sqlite3_preupdate_old, sqlite3_preupdate_count,
sqlite3_preupdate_depth, sqlite3_preupdate_new &
sqlite3_preupdate_blobwrite.
11 May 2021
- Verified the code works against SQLite v3.35.5.
2 April 2021
- Verified the code works against SQLite v3.35.3.
v1.27 (13 March 2021)
- Verified the code works against SQLite v3.35.0
v1.26 (30 January 2021)
- Updated copyright details.
- Verified the code works against SQLite v3.34.1
v1.25 (5 December 2020)
- Updated the code to compile against SQLite 3.34.0. Added support for
sqlite3_txn_state.
20 August 2020
- Verified the code works against SQLite v3.33.0.
v1.24 (14 June 2020)
- Verified the code works against SQLite v3.32.2.
- Made more methods nodiscard and constexpr.
- Fixed some compile problems when using VS 2019 Preview.
- Fixed a bug in CColumnIterator::operator> and
CRowsetIterator::operator>.
v1.23 (24 May 2020)
- Verified the code works against SQLite v3.32.0.
v1.22 (16 May 2020)
- Fixed compiler warnings when compiled using C++ 17
- Verified the code works against SQLite v3.31.1.
- Fixed more Clang-Tidy static code analysis warnings in the code.
- Fixed some problems when compiling against the latest version of
winsqlite3.h.
v1.21 (25 January 2020)
- Updated copyright details.
- Fixed more Clang-Tidy static code analysis warnings in the code.
- Verified the code works against SQLite v3.31.0.
v1.20 (18 December 2019)
- Fixed various Clang-Tidy static code analysis warnings in the code.
v1.19 (11 October 2019)
- Updated the code to compile against SQLite 3.30.1. Added support for
sqlite3_drop_modules
v1.18 (11 July 2019)
- Verified the code works against SQLite v3.29.0
v1.17 (17 April 2019)
- Updated the code to compile against SQlite 3.28.0. Added support for
sqlite3_stmt_isexplain & sqlite3_value_frombind.
v1.16 (7 April 2019)
- Fixed some compiler warnings when compiled with Visual Studio 2019
- Verified the code works against SQLite v3.27.2
v1.15 (9 February 2019)
- Verified the code works against SQLite v3.27.1
v1.14 (20 January 2019)
- Updated copyright details.
- Updated the code to compile against SQlite 3.26.0. Added support for
sqlite3_normalized_sql.
v1.13 (29 October 2018)
- Fixed some further C++ core guidelines compiler warnings.
- Updated the code to compile against SQlite 3.25.2. Added support
for sqlite3_create_window_function.
v1.12 (30 June 2018)
- Verified the code works against SQlite 3.24.0.
v1.11 (4 June 2018)
- Updated the code to compile against SQlite 3.23.1. Added support
for sqlite3_serialize, sqlite3_deserialize, sqlite3changeset_apply,
sqlite3changeset_apply_v2, sqlite3changeset_apply_strm &
sqlite3changeset_apply_v2_strm
- Fixed a number of C++ core guidelines compiler warnings. These
changes mean that the code will now only compile on VC 2017 or later.
- Verified the code compiled cleanly against winsqlite3.h header
file in versions of the Windows SDK v10.0.17125 & v10.0.17134.
v1.10 (18 March 2018)
- Updated copyright details
- Updated the code to compile against SQlite 3.22.0. Added support
for sqlite3_value_nochange.
- Verified the code compiled cleanly against winsqlite3.h header file
in versions of the Windows SDK v10.0.17069, v10.0.17083, v10.0.17095 &
v10.0.17111.
- Thoroughly reviewed all classes to ensure they had move constructors and
move operator= as necessary
- All asserts in the code are now only active in debug builds
v1.09 (28 December 2017)
- Updated the code to compile against SQLite 3.21.0. No additional changes had to be made to
code to support this new version.
- Verified the code compiled cleanly against winsqlite3.h header file in versions of the
Windows SDK v10.0.16257, v10.0.16278, v10.0.16288, v10.0.16299, v10.0.17025 & v10.0.17061.
v1.08 (20 August 2017)
- Updated the code to compile against SQLite 3.20.0. Added support for
sqlite3_set_last_insert_rowid, sqlite3_prepare_v3, sqlite3_prepare16_v3,
sqlite3_bind_pointer & sqlite3_value_pointer.
- Updated the demo app to consistently use prefix increment for iterators
in for loops.
- Updated the demo app to compile cleanly when
SQLITE3WRAPPERS_NO_WCHAR_T_SUPPORT is defined.
- Updated the code to compile cleanly with the winsqllite3.h header file
in versions of the Windows SDK v10.0.10586, v10.0.14393, v10.0.15063 &
v10.0.16232.
v1.07 (29 April 2017)
- Updated the code to compile cleanly using /permissive-.
v1.06 (18 March 2017)
- Updated copyright details.
- Updated the code to compile against SQLite 3.17.0. Added support for sqlite3_expanded_sql,
sqlite3_trace_v2, sqlite3_set_authorizer & sqlite3_system_errno.
- Updated the code to clean compile in VC 2017.
- Removed support for the deprecated APIs: sqlite3_trace & sqlite3_profile.
- Replaced NULL with nullptr throughout the code. This does mean that VC 2010
is now the minimum compiler required.
v1.05 (25 March 2016)
- Updated copyright details.
- Updated the code to clean compile in VC 2013 & VC 2015
- Addition of a "SQLITE3WRAPPERS_WIN32_INCLUSION" pre-processor
define which if set will cause SQLite3 to be pulled in via a #include <winsqlite\winsqlite3.h>.
This allows SQLiteWrappers to be compiled against the Windows 10 DLL version
of SQLite. Please note that to use this you must use the Windows 10.0.10586.0
SDK. Note that even when you do this you will probably get import errors trying
to link your code. This is because the version of SQLite included in Windows
10 is 3.8.8.3 and the winsqlite3.h header file included in the Windows SDK does
not have the correct defines in it to allow you to specify the __stdcall calling
convention when linking to the DLL. You can change the calling convention on
the translation unit which includes SQLiteWrappers.h in your application by
using the __stdcall (/Gz) compiler setting and then your code should link properly.
A checkin was done in March 2015 to the SQLite codebase which addresses this
and you should expect to see this fix in the next release of the Windows 10
SDK. For the moment because of this issue, it is still easiest to just add the
SQLite amalgamation files to your Visual Studio project to pull in support for
SQLite3.
- Updated the code to compile against SQLite 3.11.1. Added support for sqlite3_close_v2,
sqlite3_malloc64, sqlite3_realloc64, sqlite3_bind_blob64, sqlite3_bind_text64,
sqlite3_bind_zeroblob64, sqlite3_value_subtype, sqlite3_value_dup, sqlite3_value_free,
sqlite3_stmt_scanstatus, sqlite3_stmt_scanstatus_reset, sqlite3_db_cacheflush,
sqlite3_snapshot_get & sqlite3_snapshot_open
v1.04 (23 February 2013)
- Updated copyright details.
- Added support to the CStatement::Bind* methods to support binding by named
parameter. Thanks to "Prepost" for suggesting this nice addition.
v1.03 (21 July 2012)
- SQLITE3WRAPPERS_WCHAR_T_SUPPORT preprocessor value has been removed and
been replaced with the opposite which is SQLITE3WRAPPERS_NO_WCHAR_T_SUPPORT.
By default the classes will now support wchar_t string values unless the new
SQLITE3WRAPPERS_NO_WCHAR_T_SUPPORT is defined.
- Removed unnecessary reinterpret_cast's from the sample app.
v1.02 (7 July 2012)
- const and const_reverse iterators have been added to CRow class.
- CRow column iterators now support std::distance
- Fixed a number of /analyze compiler warnings
- Classes now support a SQLITE3WRAPPERS_WCHAR_T_SUPPORT preprocessor define
which treats all UTF16 strings as wchar_t* instead of void*. This can help avoid
client code needing to do casts where it has a wchar_t data type defined. It
also can help find errors where you pass UTF8 or narrow strings to a method
which requires a UTF16 string. These checks can be made more useful if you are
using the /Zc:wchar_t VC compiler setting.
- CStatement::Bind and Bind16 methods which take UTF8 and UTF16 strings now
use a default value for the length parameter
v1.01 (28 June 2012)
- CValue methods have been made const where appropriate
- CStatement methods have been made const where appropriate
- Implemented a CStatement::ExecRowset method which provides STL iterator
accessor to the returned rowset. The class returned from ExecRowset is SQLite3::CRowset
which providess an input STL iterator to traverse though all the rows returned
by SQLite3::CStatement::Step. Each row as returned from the CRowset iterator
is represented by a SQLite3::CRow. Finally SQLite3::CRow provides a random access
STL iterator to all the columns returning a SQLite3::CValue
- Two additional methods called SQLite3::CDB::ExecRowset and SQLite3::CDB::ExecRowset16
are provided which encapsulate SQLite3::CStatement::ExecRowset which do not
require any parameter binding. The output parameter from these two methods is
a SQLite3::CRowset2 which contains an SQLite3::CStatement as a member variable.
- Addition of a "SQLITE3WRAPPERS_LOCAL_INCLUSION" pre-processor
define which if set will cause SQLite3 to be pulled in via a #include "SQLite3.h"
instead of via #include <SQLite3.h>. This allows SQLiteWrappers to be
included in projects which statically compile to SQLite3 from the same directory
as your application code.
v1.0 (13 June 2012)